Programming Examples
Arduino program to interface DHT 22 sensor and find the Humidity Temperature and Heat index
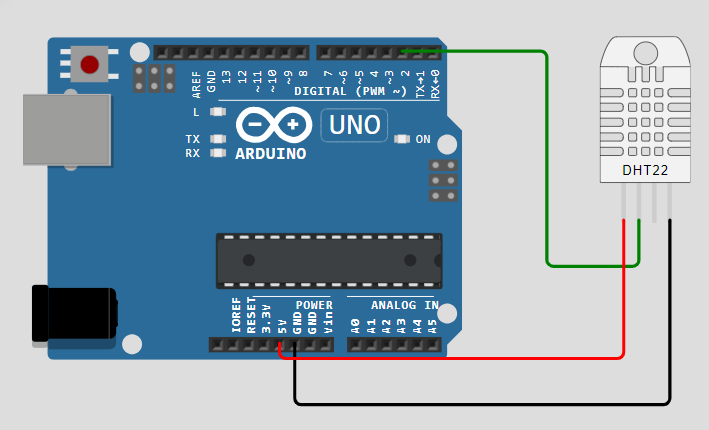
Arduino program to interface a DHT (Digital Humidity and Temperature) sensor with an Arduino, you typically use a DHT11 or DHT22 sensor. Below is a step-by-step guide to connect and program a DHT sensor with an Arduino:
Materials Needed
Arduino board (e.g., Uno, Mega, Nano)
DHT sensor (DHT11 or DHT22)
Breadboard and jumper wires
10k ohm resistor (optional for DHT22, necessary for some DHT11 modules)
Wiring the DHT Sensor
DHT Sensor Pinout:
DHT11: Usually has 3 or 4 pins: VCC, Data, GND (and NC for some modules).
DHT22: Similar to DHT11 but with better accuracy and range.
Connections:
VCC: Connect to the 5V pin on the Arduino.
GND: Connect to the GND pin on the Arduino.
Data: Connect to a digital pin on the Arduino (e.g., pin 2).
Pull-up Resistor (if needed): Connect a 10k ohm resistor between the Data pin and VCC.
Arduino Code
Install the DHT Library:
Open the Arduino IDE.
Go to Sketch > Include Library > Manage Libraries.
Search for "DHT sensor library" by Adafruit and install it.
Also, install the "Adafruit Unified Sensor" library if prompted.
Solution
#include <DHT.h>
DHT dht(2, DHT11);
void setup()
{
Serial.begin(9600);
dht.begin();
}
void loop()
{
delay(2000);
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
float heatIndex = dht.computeHeatIndex(temperature, humidity, false);
// Print the results
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" *C\t");
Serial.print("Heat index: ");
Serial.print(heatIndex);
Serial.println(" *C");
}
Output/ Explanation: