Programming Examples
Arduino program to turns on LED when the button is pressed once and remains on until the button is pressed again to turn it off
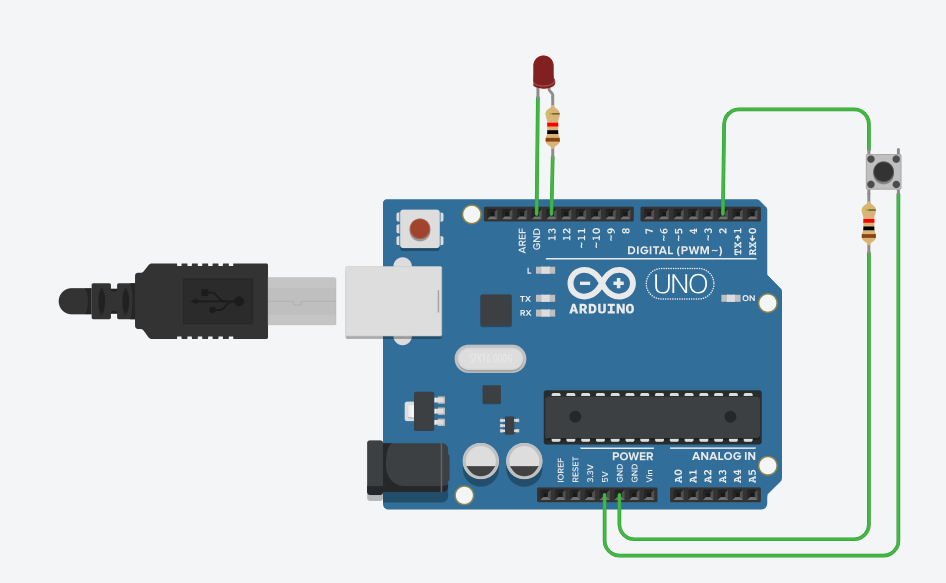
Arduino program to turns on LED when the button is pressed once and remains on until the button is pressed again to turn it off.
Output/ Explanation: